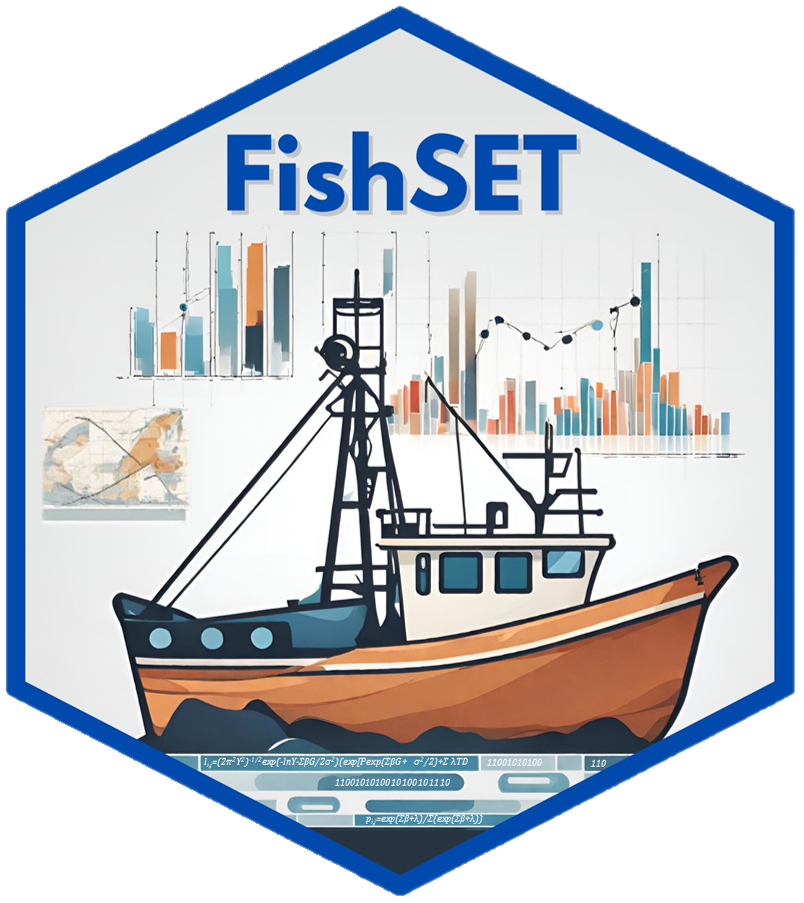
Create expected catch/expected revenue matrix
Source:R/create_expectations.R
create_expectations.Rd
Create expected catch or expected revenue matrix. The matrix is required for
the logit_c
model. Multiple user-defined matrices can be saved
by setting replace.output = FALSE
and re-running the function.
Usage
create_expectations(
dat,
project,
catch,
price = NULL,
defineGroup = NULL,
temp.var = NULL,
temporal = "daily",
calc.method = "standardAverage",
lag.method = "simple",
empty.catch = NULL,
empty.expectation = 1e-04,
temp.window = 7,
temp.lag = 0,
year.lag = 0,
dummy.exp = FALSE,
default.exp = FALSE,
replace.output = TRUE,
weight_avg = FALSE,
outsample = FALSE
)
Arguments
- dat
Primary data containing information on hauls or trips. Table in FishSET database contains the string 'MainDataTable'.
- project
String, name of project.
- catch
Variable from
dat
containing catch data.- price
Optional, variable from
dat
containing price/value data. Price is multiplied againstcatch
to generated revenue. If revenue exists indat
and you wish to use this revenue instead of price, thencatch
must be a vector of 1 of length equal todat
. Defaults toNULL
.- defineGroup
Optional, variable from
dat
that defines how to split the fleet. Defaults to treating entire dataframedat
as a fleet.- temp.var
Optional, temporal variable from
dat
. Set toNULL
if temporal patterns in catch should not be considered.- temporal
String, choices are
"daily"
or"sequential"
. Should time, iftemp.var
is defined, be included as a daily timeline or sequential order of recorded dates. For daily, catch on dates with no record are filled withNA
. The choice affects how the rolling average is calculated. If temporal is daily then the window size for average and the temporal lag are in days. If sequential, then averaging will occur over the specified number of observations, regardless of how many days they represent.- calc.method
String, how catch values are average over window size. Select standard average (
"standardAverage"
), simple lag regression of means ("simpleLag"
), or weights of regressed groups ("weights"
)- lag.method
String, use regression over entire group (
"simple"
) or for grouped time periods ("grouped"
).- empty.catch
String, replace empty catch with
NA
,0
, mean of all catch ("allCatch"
), or mean of grouped catch ("groupCatch"
).- empty.expectation
Numeric, how to treat empty expectation values. Choices are to not replace (
NULL
) or replace with 0.0001 or 0.- temp.window
Numeric, temporal window size. If
temp.var
is notNULL
, set the window size to average catch over. Defaults to 14 (14 days iftemporal
is"daily"
).- temp.lag
Numeric, temporal lag time. If
temp.var
is notNULL
, how far back to lagtemp.window
.- year.lag
If expected catch should be based on catch from previous year(s), set
year.lag
to the number of years to go back.- dummy.exp
Logical, should a dummy variable be created? If
TRUE
, output dummy variable for originally missing value. IfFALSE
, no dummy variable is outputted. Defaults toFALSE
.- default.exp
Whether to run default expectations. Defaults to
FALSE
. Alternatively, a character string containing the names of default expectations to run can be entered. Options include "recent", "older", "oldest", and "logbook". The logbook expectation is only run ifdefineGroup
is used. "recent" will not includedefineGroup
. Settingdefault.exp = TRUE
will include all four options. See Details for how default expectations are defined.- replace.output
Logical, replace existing saved expected catch data frame with new expected catch data frame? If
FALSE
, new expected catch data frames appended to previously saved expected catch data frames. Default isTRUE
. IfTRUE
- weight_avg
Logical, if
TRUE
then all observations for a given zone on a given date will be included when calculating the mean, thus giving more weight to days with more observations in a given zone. IfFALSE
, then the daily mean for a zone will be calculated prior to calculating the mean across the time window.- outsample
Logical, if
TRUE
then generate expected catch matrix for out-of-sample data. IfFALSE
generate for main data table. Defaults tooutsample = FALSE
Value
Function saves a list of expected catch matrices to the FishSET database
as projectExpectedCatch
. The list includes
the expected catch matrix from the user-defined choices, recent fine grained
information, older fine grained information, oldest fine grained information,
and logbook level information. Additional expected catch cases can be added
to the list by specifying replace.output = FALSE
. The list is
automatically saved to the FishSET database and is called
in make_model_design
. The expected catch output does not need
to be loaded when defining or running the model.
newGridVar, newDumV
Details
Function creates an expectation of catch or revenue for alternative
fishing zones (zones where they could have fished but did not). The output is
saved to the FishSET database and called by the make_model_design
function. create_alternative_choice
must be called first as observed
catch and zone inclusion requirements are defined there.
The primary choices
are whether to treat data as a fleet or to group the data (defineGroup
)
and the time frame of catch data for calculating expected catch. Catch is averaged
along a daily or sequential timeline (temporal
) using a rolling average.
temp.window
and temp.lag
determine the window size and temporal
lag of the window for averaging. Use temp_obs_table
before using
this function to assess the availability of data for the desired temporal moving
window size. Sparse data is not suited for shorter moving window sizes. For very
sparse data, consider setting temp.var
to NULL
and excluding
temporal patterns in catch.
Empty catch values are considered to be times of no fishing activity. Values
of 0 in the catch variable are considered times when fishing activity occurred
but with no catch. These points are included in the averaging and dummy creation
as points in time when fishing occurred.
Four default expected catch cases will be run:
recent: Moving window size of two days. In this case, there is no grouping, and catch for entire fleet is used.
older: Moving window size of seven days and lag of two days. In this case, vessels are grouped (or not) based on
defineGroup
argument.oldest: Moving window of seven days and lag of eight days. In this case, vessels are grouped (or not) based on
defineGroup
argument.logbook: Moving window size of 14 days and lag of one year, seven days. Only used if fleet is defined in
defineGroup
.
Examples
if (FALSE) {
create_expectations(pollockMainDataTable, "pollock", "OFFICIAL_TOTAL_CATCH_MT",
price = NULL, defineGroup = "fleet", temp.var = "DATE_FISHING_BEGAN",
temporal = "daily", calc.method = "standardAverage", lag.method = "simple",
empty.catch = "allCatch", empty.expectation = 0.0001, temp.window = 4,
temp.lag = 2, year.lag = 0, dummy.exp = FALSE, replace.output = FALSE,
weight_avg = FALSE, outsample = FALSE
)
}